[ .NET, WinForms, HTTP ]
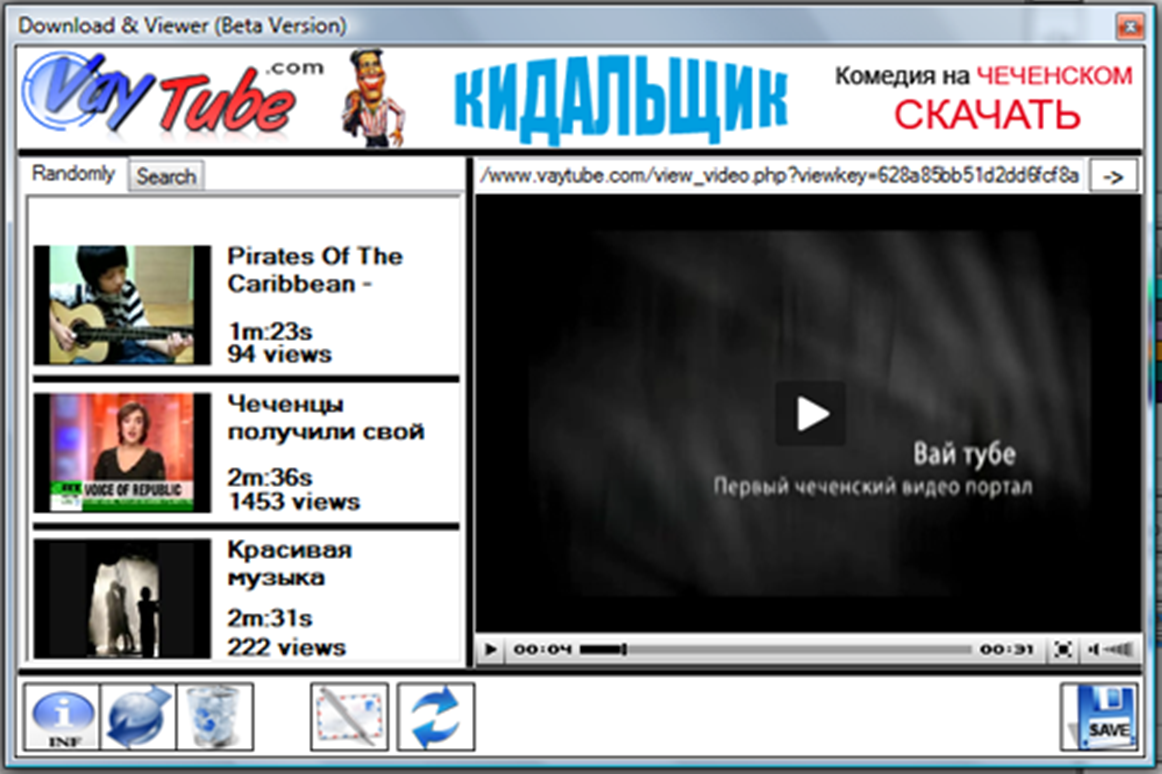
About 15 years ago, during my time as a university student and freelancer, I was given the responsibility to build a windows client for a popular video tube platform.
The resulting app was designed to provide users with an optimal viewing experience, complete with features that catered to their every need.
The app’s search function was one of its most critical components, as it allowed users to find videos quickly and easily. By entering a search term, users could get relevant results that included video titles, thumbnails, and other essential details.
To make searching even more efficient, the app included filters that allowed users to narrow down their search by video length, upload date, and category.
Once a user found a video they wanted to watch, they could do so within the app’s fast and responsive video player. This feature also gave users the ability to adjust video quality to suit their internet speed. Additionally, users could leave comments and ratings on the videos, helping others make informed decisions on which videos to watch.
Perhaps one of the app’s most popular features was the ability to download videos for offline viewing. This feature was especially useful for users with limited internet access, ensuring they could enjoy their favorite videos even when they were not connected to the internet.
Unfortunately this project was proprietory and the server has long been shut down.