[ .NET, Console, C#, System.IO ]
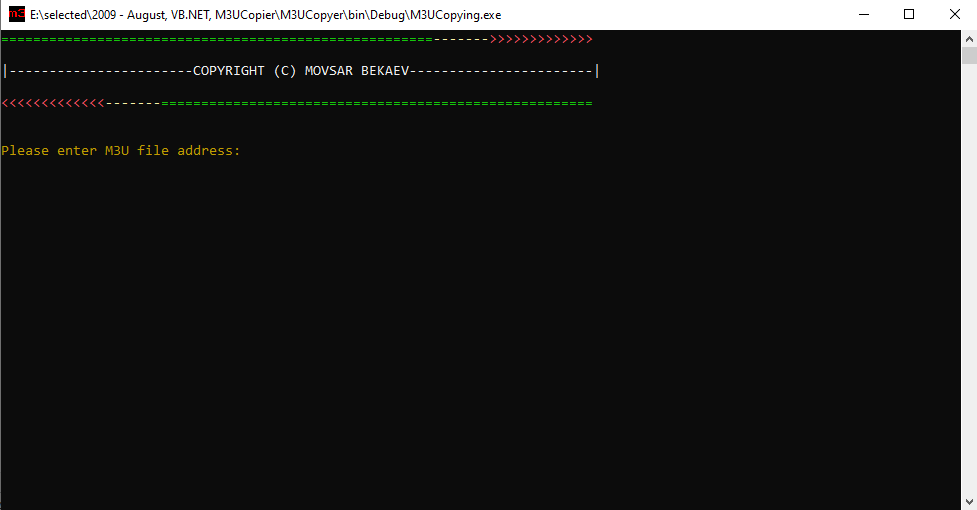
In this article, we will examine a code snippet that copies files from an M3U file to a designated folder. We will discuss its functionality, how it works, and its benefits. It’s a simple Windows Console app that I wrote when dealing with some playlists trying to organize music files on my local machine.
The code begins by checking if the arguments passed to it are less than one. If this is true, the program will prompt the user to enter the path of the M3U file and the destination folder where the files will be copied. This is done through the Console class of the System namespace.
After prompting the user for input, the code then reads the contents of the M3U file using a StreamReader class. The StreamReader class is used to read characters from a stream in a specified encoding. In this case, the encoding used is 1251. The next section of the code extracts the file names from the M3U file and adds them to a collection called fNamesCol. This is done using a while loop that reads each line of the M3U file until it reaches the end of the file. Within this loop, there is an if statement that checks if the current line is the first line of the file. If it is, it reads the line and adds it to the collection. If it is not, it skips the line.
Once the file names have been extracted, the code then checks if there are any files in the collection. If there are, it then copies each file from its source location to the destination folder. This is done using a foreach loop that iterates through each file in the collection. Within this loop, a new FileInfo object is created for each file, and the File.Copy method is called to copy the file from its source location to the destination folder. After all the files have been copied, the code then displays a message to indicate that the process is complete. The code then waits for the user to press enter before opening the destination folder using the Process.Start method.
try
{
Console.ForegroundColor = ConsoleColor.DarkYellow;
Console.WriteLine("Please enter the path of the M3U file:");
Console.ForegroundColor = ConsoleColor.Yellow;
var m3uFilePath = Console.ReadLine();
Console.ForegroundColor = ConsoleColor.DarkYellow;
Console.WriteLine("Please enter the path of the collection where you want to copy the files:");
Console.ForegroundColor = ConsoleColor.Yellow;
var collectionPath = Console.ReadLine();
var fileNamesCollection = new List<string>();
var i = 0;
using (var streamReader = new StreamReader(m3uFilePath, System.Text.Encoding.GetEncoding(1251)))
{
streamReader.ReadLine(); // skip the first line
while (!streamReader.EndOfStream)
{
if (i == 1)
{
var fileName = streamReader.ReadLine();
if (fileName.Substring(1, 1) != ":")
{
fileName = $"{m3uFilePath.Substring(0, 3)}{fileName}";
}
fileNamesCollection.Add(fileName);
i = 0;
}
else
{
streamReader.ReadLine();
i++;
}
}
}
if (fileNamesCollection.Count > 0)
{
if (!collectionPath.EndsWith("\\"))
{
collectionPath += "\\";
}
foreach (var fileName in fileNamesCollection)
{
var file = new FileInfo(fileName);
Console.WriteLine(fileName);
File.Copy(file.FullName, $"{collectionPath}{file.Name}", true);
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("OK!");
Console.ForegroundColor = ConsoleColor.DarkYellow;
}
Console.ReadLine();
}
Console.WriteLine("Done!");
Console.ReadLine();
System.Diagnostics.Process.Start("explorer", collectionPath);
}
catch (FileNotFoundException)
{
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine("The M3U file cannot be found!");
System.Threading.Thread.Sleep(1000);
}
catch (Exception ex)
{
Console.ForegroundColor = ConsoleColor.Red;
Console.WriteLine($"An error occurred: {ex.Message}");
System.Threading.Thread.Sleep(1000);
}
In summary, this code snippet automates the process of copying files from an M3U file to a designated folder. It achieves this by reading the contents of the M3U file, extracting the file names, and copying them to the destination folder.
Download: M3UCopying
Leave a reply