[ .NET, WinForms, System.Text ]
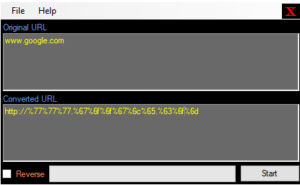
In the realm of hacking, it is often necessary to conceal the true URL, preventing users from recognizing its text before clicking. One way to accomplish this is through the use of URL encoding, a process that converts a URL into a code representation that can be easily read and understood by machines, but not by humans.
URL encoding is a method that allows special characters within a URL, such as ampersands, spaces, and question marks, to be replaced with hexadecimal values, making the URL easier to process and manipulate by automated tools. By encoding URLs, hackers can evade detection and gain access to sensitive data that would otherwise be off-limits.
In the hands of a skilled hacker, this technique can be used to exploit vulnerabilities in a website’s code, steal user information, and carry out other malicious activities. As such, it is important for website owners and developers to be aware of URL encoding and take steps to protect their sites from potential attacks.
It can be used to convert URLs to code representation and back to normal. It’s quite simple and most of the work is done in this simple replacement method:
Private Sub SymToCod()
Dim specialChars() As Char = "-./\:;?#@'+!<>"
Dim encodedChars() As String = {"%2D", ".", "/", "\", ":", ";", "?", "#", "@", "'", "+", "!", "<", ">"}
Dim result As New StringBuilder("http://")
For Each c As Char In rtbInput.Text
If specialChars.Contains(c) Then
result.Append(encodedChars(Array.IndexOf(specialChars, c)))
Else
For j = 0 To 35
If syms(j).Contains(c) Then
result.Append(Microsoft.VisualBasic.Left(syms(j), 3))
Exit For
End If
Next
End If
progressMain.Value += 1
Next
rtbOutput.Text = result.ToString()
End Sub
- The code defines two arrays: specialChars and encodedChars. The specialChars array contains the characters that need to be replaced, while encodedChars contains the encoded versions of these characters.
- The input string is taken from a rtbInput control, and the resulting encoded string is stored in a new StringBuilder object called “result.”
- For each character in the input string, the code checks if it is a special character. If it is, the code replaces it with its corresponding encoded character from the encodedChars array. If not, it moves on to the next step.
- The code then checks if the character matches any of the characters in the syms array. If it does, the corresponding three-character code is appended to the result string.
- A progress bar called progressMain is also incremented with each character processed.
- Finally, the resulting encoded string is displayed in another rtbOutput control.
Overall, this code is useful for anyone who needs to encode special characters in URLs to ensure that they are transmitted correctly. I wrote this app more than a decade ago, now there are libraries for each programming language that makes it easy to use URL encoding, yet it might still be valuable for someone to peek into the details and see how it works.
Download: URLHiding
Leave a reply